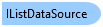
class Product { public int ProductID { get; set; } public string ProductName { get; set; } } class FastListDataSource : IListDataSource { private readonly System.Collections.Generic.IList < Product > _list; private readonly System.Data.IDataReader _reader; public FastListDataSource(System.Collections.Generic.IList < Product > list) { _list = list; _reader = FastMember.ObjectReader.Create(_list); } public object GetValue(string fieldName, int ordinal) { var index = fieldName.IndexOf('.'); if (index == -1) return _reader.GetValue(ordinal); var fields = fieldName.Split('.'); var target = _reader.GetValue(ordinal); for (int i = 1; i != fields.Length; i++) target = FastMember.ObjectAccessor.Create(target)[fields[i]]; return target; } public int GetOrdinal(string fieldName) { var index = fieldName.IndexOf('.'); if (index == -1) return _reader.GetOrdinal(fieldName); return _reader.GetOrdinal(fieldName.Substring(0, index)); } public System.Collections.IList List => (System.Collections.IList)_list; public System.Data.IDataReader DataReader => _reader; }
class Product { public int ProductID { get; set; } public string ProductName { get; set; } } class FastListDataSource : IListDataSource { private readonly System.Collections.Generic.IList < Product > _list; private readonly System.Data.IDataReader _reader; public FastListDataSource(System.Collections.Generic.IList < Product > list) { _list = list; _reader = FastMember.ObjectReader.Create(_list); } public object GetValue(string fieldName, int ordinal) { var index = fieldName.IndexOf('.'); if (index == -1) return _reader.GetValue(ordinal); var fields = fieldName.Split('.'); var target = _reader.GetValue(ordinal); for (int i = 1; i != fields.Length; i++) target = FastMember.ObjectAccessor.Create(target)[fields[i]]; return target; } public int GetOrdinal(string fieldName) { var index = fieldName.IndexOf('.'); if (index == -1) return _reader.GetOrdinal(fieldName); return _reader.GetOrdinal(fieldName.Substring(0, index)); } public System.Collections.IList List => (System.Collections.IList)_list; public System.Data.IDataReader DataReader => _reader; }