This scenario requires the below lines of code.
C# |
Copy Code
|
// build key-value dictionary
var dct = new Dictionary<int, string>();
foreach (var country in GetCountryNames())
{
dct[dct.Count] = country;
}
// assign dictionary to column
var c = _flexEdit.Columns["CountryID"];
c.ValueConverter = new ColumnValueConverter(dct);
c.HorizontalAlignment = HorizontalAlignment.Left;
|
The code starts by building a dictionary that maps country ID values (integers) to country names (strings).
It then uses the dictionary to build a ColumnValueConverter and assigns the converter to the column's ValueConverter property as in the previous examples.
The user will be able to select any countries present in the dictionary, and will not be able to enter any unmapped values.
Finally, the code sets the column alignment to left. Since the column actually contains integer values, it is aligned to the right by default. But since we are now displaying names, left alignment is a better choice here.
The image below shows the appearance of the editor while selecting a value from a list. Notice how the editor supports smart auto-completion, so as the user types “Ger,” the dropdown automatically selects the only valid option “Germany” (and not “Guatemala,” then “Eritrea,” then “Romania”).
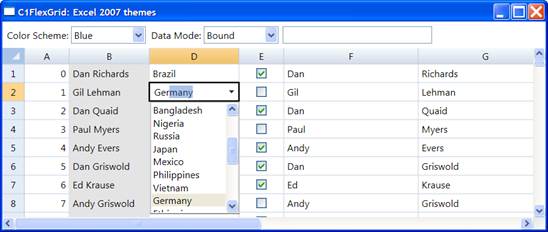
This scenario requires the below lines of code.
C# |
Copy Code
|
public void DataMapColumn()
{
//Hide Country Column
var country_col = _flexEdit.Columns["Country"];
country_col.IsVisible = false;
//Make the CountryID column read only
var c = _flexEdit.Columns["CountryID"];
c.IsReadOnly = true;
//Display Country name instead of CountryID
//using GridDataMap
var columnMap = new GridDataMap();
columnMap.ItemsSource = view;
columnMap.SelectedValuePath = "CountryID";
columnMap.DisplayMemberPath = "Country";
c.DataMap = columnMap;
|
The code uses GridDataMap class that maps Country ID key values (integers) to Country Names (strings) using SelectedValuePath and DisplayMemberPath properties.
ItemsSource property is used to get the collection to map. The image below shows the appearance of the editor while selecting a value from a list.
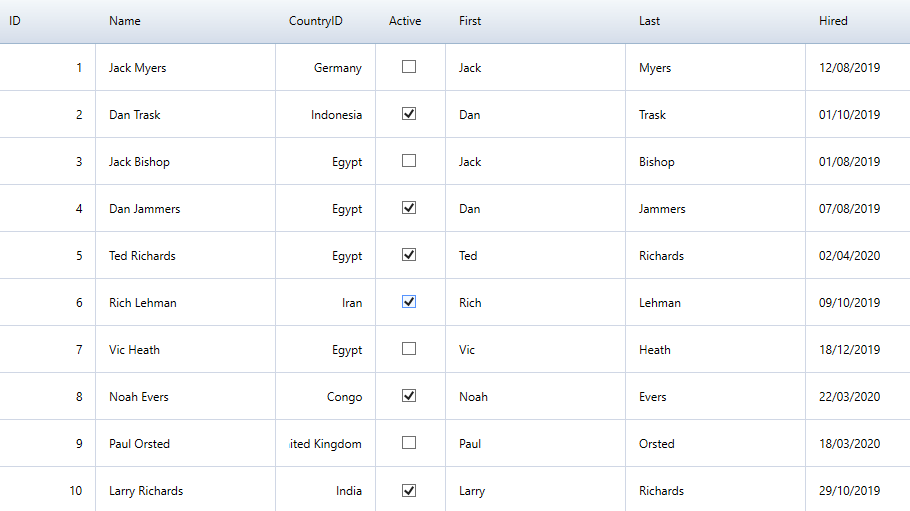