Download an Excel file in the browser in an ASP.NET MVC Core project
Background:
When using our C Sharp .NET Excel API, GcExcel, in an MVC some users may want an Excel file to be downloaded within the Browser and not to their local drive. Users can accomplish this by creating a response with headers and writing the stream of the workbook to it.
Getting Started:
We must first create a memory stream and then using GcExcel’s save method we will save the created workbook to a stream. Be sure to reset the stream to its original position after saving:
Workbook workbook = new Workbook();
workbook.Worksheets[0].Range["A1"].Value = "Hello Word!";
// Create a stream
MemoryStream stream = new MemoryStream();
// Save the workbook to the file stream
workbook.Save(stream);
// After you save to the stream, you need to reset the stream back to the origin at position 0
stream.Seek(0, SeekOrigin.Begin);
Then we will write a response with headers and write the memory stream to the response:
Workbook workbook = new Workbook();
workbook.Worksheets[0].Range["A1"].Value = "Hello Word!";
// Create a stream
MemoryStream stream = new MemoryStream();
// Save the workbook to the file stream
workbook.Save(stream);
// After you save to the stream, you need to reset the stream back to the origin at position 0
stream.Seek(0, SeekOrigin.Begin);
// Response to the user
Response.ContentType = "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet";
Response.Headers["content-disposition"] = "attachment; filename=HelloWord.xlsx";
Response.Body.WriteAsync(stream.ToArray());
After applying this code logic and running the MVC (ASP.NET) project, the excel file is downloaded to the browser like so:
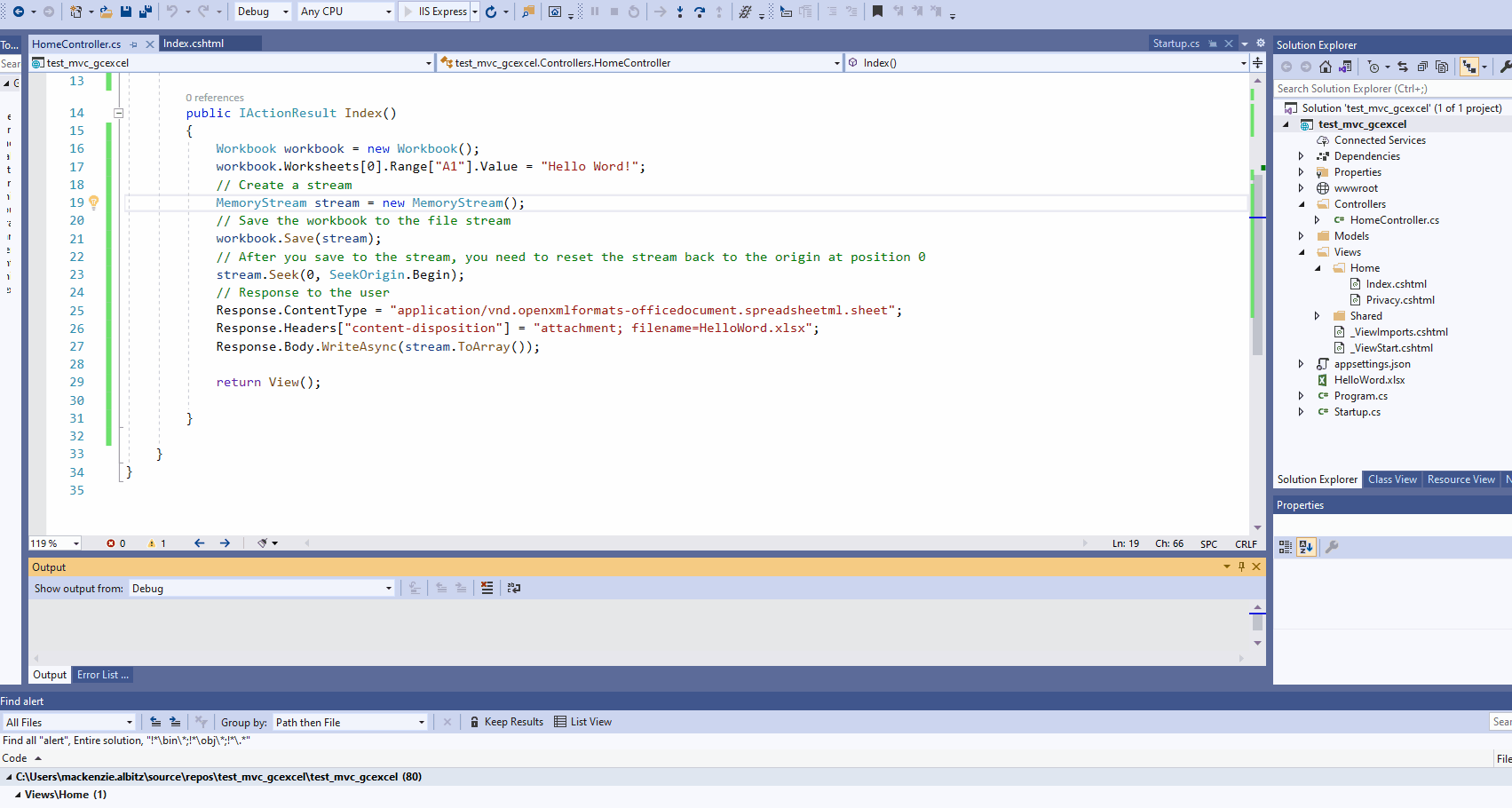
Tags:
Mackenzie Albitz