Background:
To export one worksheet to an Excel file, instead of the entire workbook of a SpreadJS instance, developers need to create a temporary workbook that will only store the worksheet that is needed to be exported. Then they can save the temporary workbook as an Excel file, only containing the one worksheet.
Steps to Complete:
1. Save and load worksheet’s json to a temporary workbook
2. Save the temporary workbook as an Excel file
Getting Started:
Step 1: Send the worksheet’s json to a temporary workbook
SpreadJS’s API library has a toJSON method that will save the worksheet object to a JSON string. Once the worksheet is a JSON string, create an empty, temporary workbook instance to load the JSON data to using the fromJSON method.
In this example we are exporting the active sheet of the current SpreadJS instance.
document.getElementById('saveExcel').onclick = function() {
// 1.1) Store the active sheet's JSON data
var json = spread.getActiveSheet().toJSON();
// 1.2) Create temporary workbook
var tempSpread = new GC.Spread.Sheets.Workbook(document.createElement("DIV"));
tempSpread.sheetCount=1;
// 1.3) Load the JSON data from the activesheet to the temporary workbook’s sheet
tempSpread.getActiveSheet().fromJSON(json);
};
Step 2: Save the temporary workbooks JSON to Excel file
Next, use the workbooks toJSON method and Excel IO’s Save method to export the temporary instance as an Excel file:
document.getElementById('saveExcel').onclick = function() {
// 1.1) Store activesheets JSON in variable
var json = spread.getActiveSheet().toJSON();
console.log(json)
// 1.2) Create temporary workbook
var tempSpread = new GC.Spread.Sheets.Workbook(document.createElement("DIV"));
tempSpread.sheetCount=1;
// 1.3) Implement the JSON data from the activesheet to the temporary workbook’s sheet
tempSpread.getActiveSheet().fromJSON(json);
// 2.1) Store temporary sheet as JSON data
var newJson = tempSpread.toJSON();
// 2.2) Save temporary workbook as Excel file
excelIo.save(newJson, function(blob) {
saveAs(blob, "myFile.xlsx");
}, function(e) {
// process error
console.log(e);
});
};
Outcome:
After applying the code logic shared here only the activesheet from within the workbook will be exported:
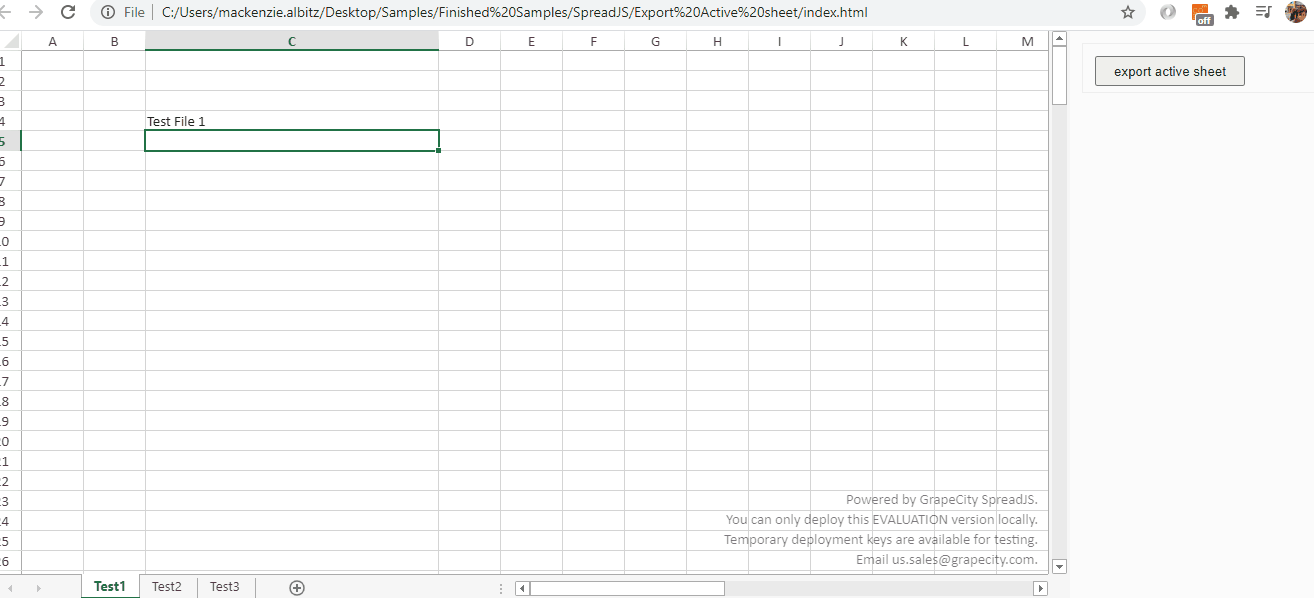
Here is a link to a live sample: https://codesandbox.io/s/sjs-js-export-active-worksheet-only-m00nv?file=/index.html
Mackenzie Albitz