How to display the formatting of a cell when the cell is in edit mode in JavaScript
Background:
To display a cell’s formatting during edit mode use the setEditorValue method to customize the cells editor value.
Steps to Complete:
1. Create a cell with a custom editor value
2. Set the editor value to display the same value and formatting
Getting Started:
Step 1: Create a cell with a custom editor value
Use the setEditorValue method and get the cell value and formatter from the row and column indexes.
GC.Spread.Sheets.CellTypes.Text.prototype.setEditorValue = function (
editor,
value,
context
) {
var sheet = context.sheet,
row = context.row,
column = context.col;
// 1.) Get the unformatted data from the specified cell in the specified sheet area for the row and col index
var cellValue = sheet.getValue(row, column);
// 1.) Get the cell formatter for the row and col index
var formatter = sheet.getFormatter(row, column);
};
Step 2: Set the cells editor value
Set the editor value to display the same value and formatting by first setting the value to be equal to SpreadJS’s the GeneralFormatter class with the returned formatter of the selected cell.
Then call for the custom cell to set the editor with the value that contains the formatting of the cell.
var newForm = GC.Spread.Sheets.CellTypes.Text.prototype.setEditorValue;
GC.Spread.Sheets.CellTypes.Text.prototype.setEditorValue =
function(editor, value, context) {
var sheet = context.sheet, row = context.row, column = context.col;
var cellValue = sheet.getValue(row, column);
var formatter = sheet.getFormatter(row, column);
if (cellValue && formatter) {
value = new GC.Spread.Formatter.GeneralFormatter(formatter).format(value);
newForm.call(this, editor, value, context);
}
else {
newForm.apply(this, arguments);
}
};
Outcome:
After adding this the cell editor results with the cells value with formatting where by default SpreadJS will display the value:
This is the Cell Editor by default:
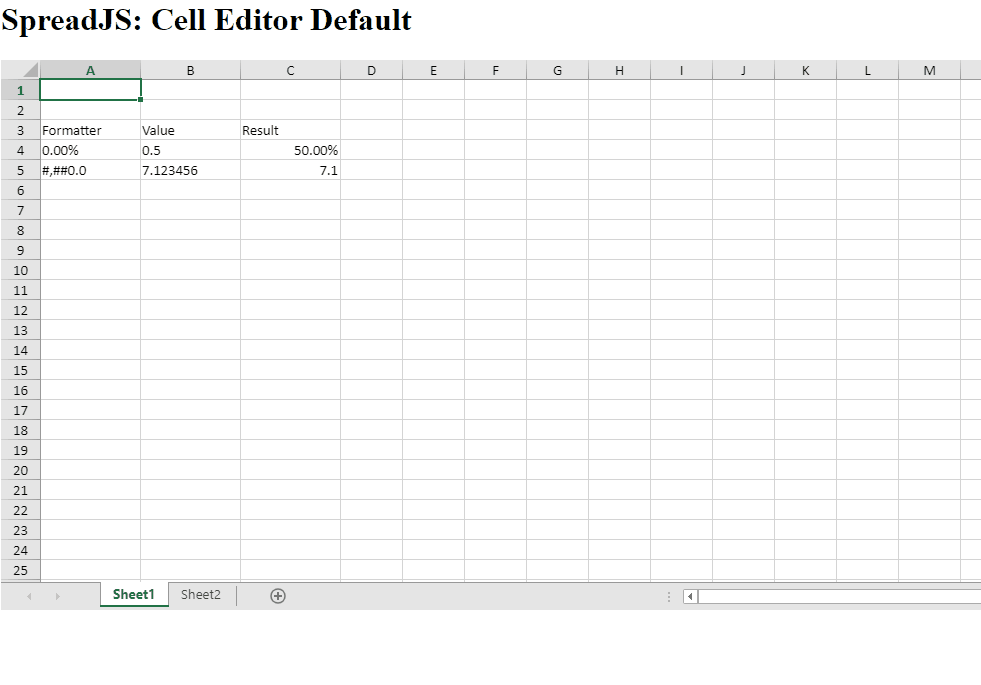
This is SpreadJS with the custom code as described in this article:
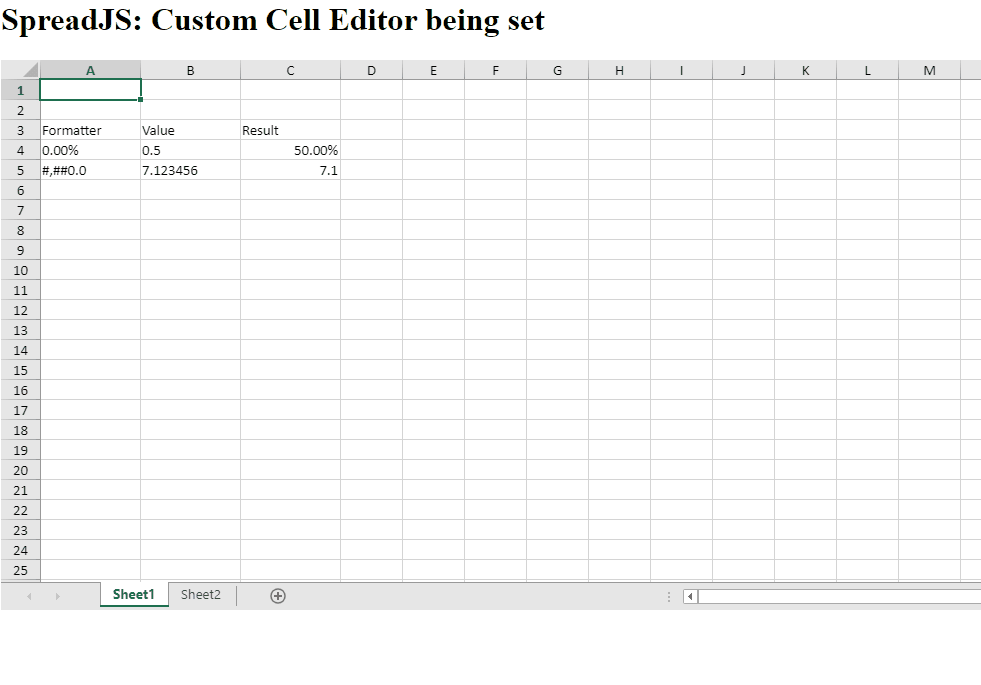
Tags:
Mackenzie Albitz