Background:
A user of SpreadJS, our Javascript spreadsheet API, can intercept when a new row or column is added to the spreadsheet instance using the RowChanging and columnChanging events.
How to accomplish:
Using SpreadJS’s Javascript spreadsheet API, the rowChanging and columnChanging events can be bound to the entire Spread instance or a specified sheet. In this example it is bound to the workbook. When adding a row or column is added these events are triggered; developers can then intercept the newly added row/column.
Here is a code snippet that will make the background color of the new row or column lightblue:
spread.bind(GC.Spread.Sheets.Events.RowChanging, function (e, args) {
var row = args.row;
var sheet = args.sheet;
console.log("Row change:" + row)
activeSheet.getRange(row, -1, 1, -1, GC.Spread.Sheets.SheetArea.viewport).backColor("lightblue");
});
spread.bind(GC.Spread.Sheets.Events.ColumnChanging, function (e, args) {
var col = args.col;
var sheet = args.sheet;
console.log("Column change:" + col)
activeSheet.getRange(-1, col, -1, 1, GC.Spread.Sheets.SheetArea.viewport).backColor("lightblue");
});
Outcome:
By applying this logic, when a new row or column is inserted, these events are triggered and set the backColor property of the cell range of the newly added row or column.
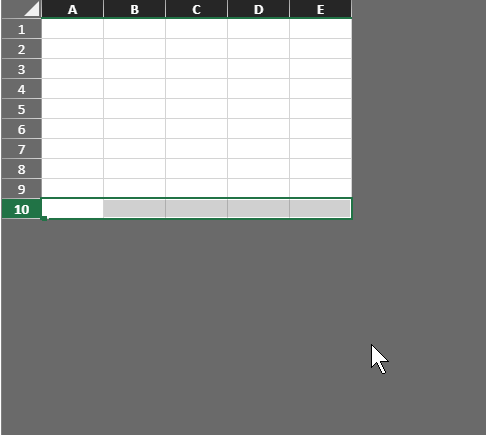
Here is a link to a live sample showing this: https://codesandbox.io/s/sjs-js-intercept-when-a-new-row-col-is-added-lkm0f?file=/index.html
Mackenzie Albitz